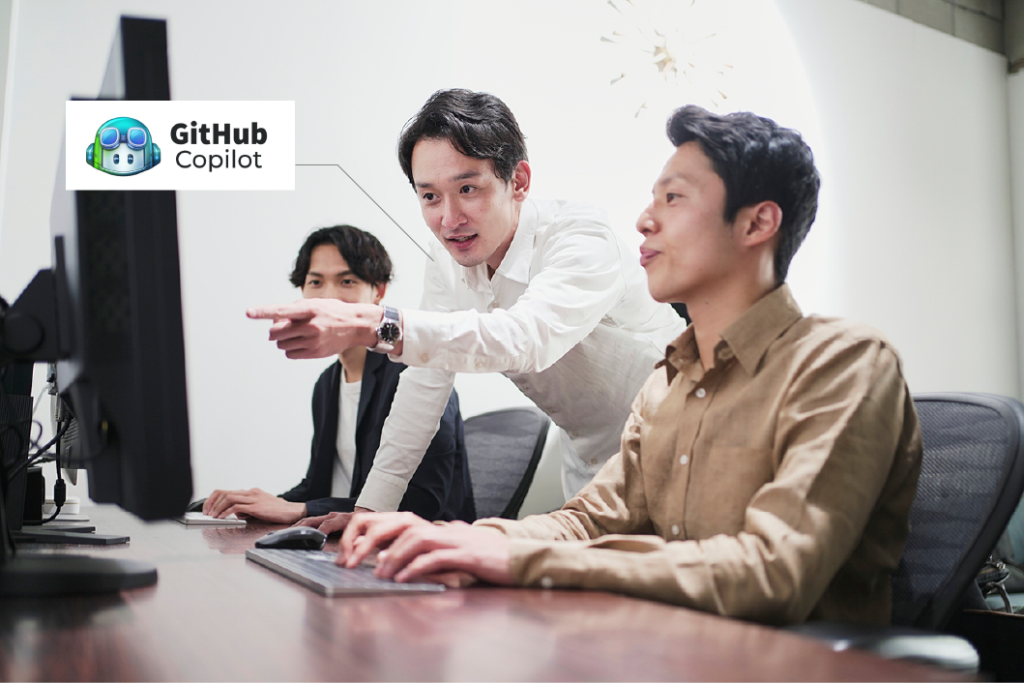
目次
GitHub Copilotとは
- GitHub Copilot とは、OpenAI Codex の技術を利用したコーディング時のオートコンプリートシステムです。
- GitHub上のGPLソースコードを沢山学習した情報を使い、プログラムのコメントや関数名の記入により的確なコード補完の補助をしてくれます。
- 但し、GitHub上のソースコードを学習して作られたGitHub Copilotを商業利用することについては、「著作権的に問題があるのではないか?」との議論もあります。
必要なもの
- GitHubアカウント
- GitHub Copilotからの招待
- 現在、Technical Preview状態なので、利用には申請が必要です。
- コードエディタ
- 現在、下記のエディタで利用できます。
- ただし、プラグイン、機能拡張のインストールが必要です。
- Visual Studio Code
- Neovim
- IntelliJ IDEA
- Visual Studio Code利用の場合
- Getting Started with GitHub Copilot in Visual Studio Code のページの内容に従い、拡張機能をインストールします。
Pythonコーディング時の補完の様子
公式サイトで表示されているPythonプログラムのサンプルを Visual Studio Code で試した様子の動画です。
Pythonコーディングの詳細
import datetime
def parse_expenses(expenses_string):
ここまで入力した時点で、関数名から機能予測をしてコードの候補を提案してくれます。
import datetime
def parse_expenses(expenses_string):
expenses = []
for expense in expenses_string.split(','):
expenses.append(float(expense))
return expenses
提案してもらった関数の内容を削除して、作成したい機能のヒントとなるコメントを記入します。
import datetime
def parse_expenses(expenses_string):
"""Parse the list of expenses and return the list of triples (date, value, currency).
Ignore lines starting with #.
Parse the date using datetime.
Example expenses_string:
2016-01-02 -34.01 USD
2016-01-03 2.59 DKK
2016-01-03 -2.72 EUR
"""
コメント入力完了で、コードを予測補完してくれます。「TABキー」をクリックすると、補完が完成します。
import datetime
def parse_expenses(expenses_string):
"""Parse the list of expenses and return the list of triples (date, value, currency).
Ignore lines starting with #.
Parse the date using datetime.
Example expenses_string:
2016-01-02 -34.01 USD
2016-01-03 2.59 DKK
2016-01-03 -2.72 EUR
"""
expenses = []
for line in expenses_string.splitlines():
if line.startswith('#'):
continue
date, value, currency = line.split()
expenses.append((datetime.datetime.strptime(date, '%Y-%m-%d'), float(value), currency))
return expenses
Pythonのモジュールが直接実行された時の分岐を記載しようとすると
import datetime
def parse_expenses(expenses_string):
"""Parse the list of expenses and return the list of triples (date, value, currency).
Ignore lines starting with #.
Parse the date using datetime.
Example expenses_string:
2016-01-02 -34.01 USD
2016-01-03 2.59 DKK
2016-01-03 -2.72 EUR
"""
expenses = []
for line in expenses_string.splitlines():
if line.startswith('#'):
continue
date, value, currency = line.split()
expenses.append((datetime.datetime.strptime(date, '%Y-%m-%d'), float(value), currency))
return expenses
if __name__ == '__main__':
それに必要なコードも補完提案してくれます。
import datetime
def parse_expenses(expenses_string):
"""Parse the list of expenses and return the list of triples (date, value, currency).
Ignore lines starting with #.
Parse the date using datetime.
Example expenses_string:
2016-01-02 -34.01 USD
2016-01-03 2.59 DKK
2016-01-03 -2.72 EUR
"""
expenses = []
for line in expenses_string.splitlines():
if line.startswith('#'):
continue
date, value, currency = line.split()
expenses.append((datetime.datetime.strptime(date, '%Y-%m-%d'), float(value), currency))
return expenses
if __name__ == '__main__':
expenses = parse_expenses('''2016-01-02 -34.01 USD
2016-01-03 2.59 DKK
2016-01-03 -2.72 EUR''')
print(expenses)
これでコード完成です。
完成したPythonプログラムの実行
- 完成したプログラムを実行します。
- Anaconda3の環境にて、実行結果が表示されます。(Anaconda3は、別途設定が必要)
PS C:\Users\ariki\Desktop\GitHub_Copilot> & C:/Users/ariki/anaconda3/python.exe c:/Users/ariki/Desktop/GitHub_Copilot/test.py
[(datetime.datetime(2016, 1, 2, 0, 0), -34.01, 'USD'), (datetime.datetime(2016, 1, 3, 0, 0), 2.59, 'DKK'), (datetime.datetime(2016, 1, 3, 0, 0), -2.72, 'EUR')]
PS C:\Users\ariki\Desktop\GitHub_Copilot>